Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 월간결산
- 파이썬 시각화
- 한빛미디어서평단
- 매틀랩
- MySQL
- python visualization
- Ga
- 딥러닝
- 리눅스
- tensorflow
- Python
- 서평
- 한빛미디어
- Linux
- SQL
- 독후감
- MATLAB
- 서평단
- 통계학
- 티스토리
- Pandas
- 텐서플로
- 시각화
- matplotlib
- Blog
- Google Analytics
- Tistory
- 블로그
- Visualization
- 파이썬
Archives
- Today
- Total
pbj0812의 코딩 일기
[PYTHON] matplotlib 으로 Parallel Categories Charts 구현하기 본문
ComputerLanguage_Program/PYTHON
[PYTHON] matplotlib 으로 Parallel Categories Charts 구현하기
pbj0812 2021. 8. 18. 02:090. 목표
- matplotlib 으로 Parallel Categories Charts 구현하기
1. plotly 의 Parallel Categories Charts
1) library 호출
import plotly.express as px
import pandas as pd
2) 데이터 생성
iris = px.data.iris()
3) 그림 그리기
fig = px.parallel_coordinates(iris, color="species_id")
fig.show()
- 결과
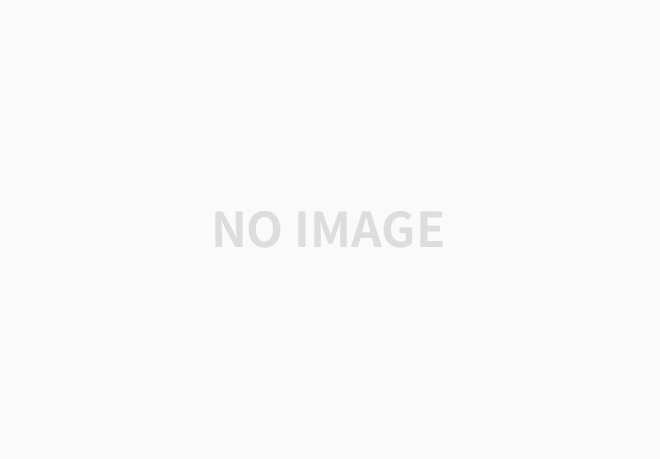
2. matplotlib 으로 구현
1) library 호출
import matplotlib.pyplot as plt
import pandas as pd
# ytick 강제 변형을 위해 사용
import numpy as np
import matplotlib.ticker as mticker
2) 모든 데이터를 백분율로 변환
iris['sepal_length_percent'] = (iris['sepal_length'] - min(iris['sepal_length'])) / (max(iris['sepal_length']) - min(iris['sepal_length'])) * 100
iris['sepal_width_percent'] = (iris['sepal_width'] - min(iris['sepal_width'])) / (max(iris['sepal_width']) - min(iris['sepal_width'])) * 100
iris['petal_length_percent'] = (iris['petal_length'] - min(iris['petal_length'])) / (max(iris['petal_length']) - min(iris['petal_length'])) * 100
iris['petal_width_percent'] = (iris['petal_width'] - min(iris['petal_width'])) / (max(iris['petal_width']) - min(iris['petal_width'])) * 100
iris['species_percent'] = (iris['species_id'] - min(iris['species_id'])) / (max(iris['species_id']) - min(iris['species_id'])) * 100
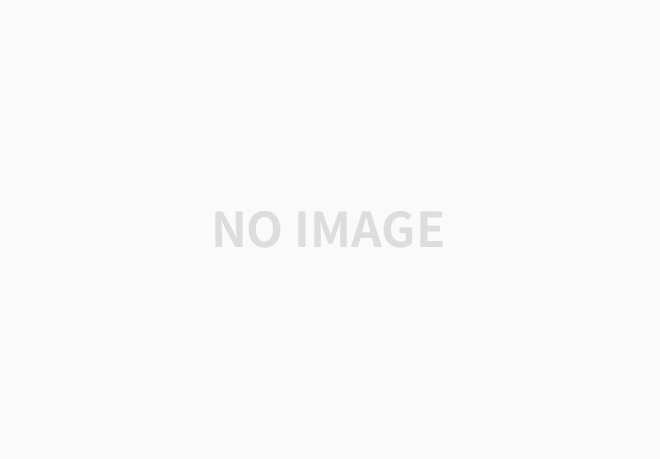
3) 사용될 변수 리스트 생성
- column_list : 그림을 그리는 데 사용될 백분율 데이터의 필드명
- column_list2 : y_ticks 강제 변환을 위해 이용될 원래의 데이터 필드명
column_list = ['sepal_length_percent', 'sepal_width_percent', 'petal_length_percent', 'petal_width_percent', 'species_percent']
column_list2 = ['sepal_length', 'sepal_width', 'petal_length', 'petal_width', 'species_id']
df_len = len(iris['species'])
4) 그림 그리기
# 도화지
# 5개 구역
fig, ax = plt.subplots(1, len(column_list))
# 그림 크기
fig.set_size_inches(15, 4)
# 피규어를 다 붙여버림
fig.subplots_adjust(wspace=0, hspace=0)
# 라벨, tick, 윤곽선 컨트롤
for i in range(len(column_list)):
# xtick 삭제
ax[i].set_xticks([])
ax[i].set_xlim([0, 1])
ax[i].set_ylim([0, 100])
# y_tick 강제 변형
ax[i].yaxis.set_major_locator(mticker.FixedLocator(ax[i].get_yticks().tolist()))
ax[i].set_yticklabels([round(i, 1) for i in np.array(ax[i].get_yticks().tolist()) / 100 * (max(iris[column_list2[i]]) - min(iris[column_list2[i]]))+ min(iris[column_list2[i]])])
# 왼쪽 윤곽선을 제외한 나머지 선들 제거
ax[i].spines['top'].set_visible(False)
ax[i].spines['bottom'].set_visible(False)
ax[i].spines['right'].set_visible(False)
# y 축 라벨
ax[i].set_ylabel(column_list2[i], rotation = 0, fontsize = 15)
# y 축 라벨 위치
ax[i].yaxis.set_label_coords(-0.1,1.02)
# 마지막 피규어는 윤곽선만 사용할 것이기에 전체길이 -1 까지
for i in range(len(column_list) - 1):
for j in range(df_len):
# species_id 데이터를 이용하여 색상 지정
if iris['species_id'][j] == 1:
color = '#ffb6c1'
elif iris['species_id'][j] == 2:
color = '#87ceeb'
else:
color = '#adff2f'
ax[i].plot([0, 1], [iris[column_list[i]][j], iris[column_list[i + 1]][j]], color = color)
- 결과
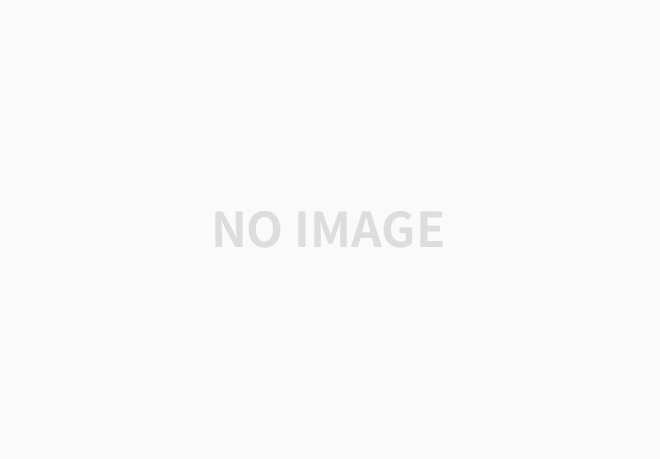
3. 참고
- Matplotlib 에서 X 축 눈금 레이블 텍스트를 회전하는 방법
- Setting the position of the `ylabel` in a matplotlib graph
'ComputerLanguage_Program > PYTHON' 카테고리의 다른 글
[PYTHON] OpenCV 를 이용한 얼굴 비율 산출기 제작 (0) | 2021.09.10 |
---|---|
[PYTHON] 두 개의 dict 내 구성요소 일치여부를 판단하기 (0) | 2021.08.29 |
[PYTHON] GridSpec 을 이용한 여러 그래프를 같이 그리기 (0) | 2021.08.17 |
[PYTHON] 태극문양 그리기 (2) | 2021.08.16 |
[PYTHON] matplotlib 으로 전단지 만들기 (0) | 2021.08.15 |